#for Python 2 #import Tkinter as tk #import ttk #for Python 3 import tkinter as tk from tkinter import ttk import platform def quit(): global tkTop tkTop.destroy() tkTop = tk.Tk() tkTop.geometry('500x400') tkLabelTop = tk.Label(tkTop, text=" http://hello-python.blogspot.com ") tkLabelTop.pack() strVersion = "running Python version " + platform.python_version() tkLabelVersion = tk.Label(tkTop, text=strVersion) tkLabelVersion.pack() strPlatform = "Platform: " + platform.platform() tkLabelPlatform = tk.Label(tkTop, text=strPlatform) tkLabelPlatform.pack() tkButtonQuit = tk.Button( tkTop, text="Quit", command=quit) tkButtonQuit.pack() lf1 = ttk.LabelFrame(tkTop, text="LabelFrame 1") lf1.pack(fill="x", expand="yes") def rbCallback(): varLabel.set("Radiobutton clicked: " + str(rbVar.get())) rbVar = tk.IntVar() rb1 = tk.Radiobutton( lf1, text="One", variable=rbVar, value=1, command=rbCallback) rb1.pack(anchor=tk.W) rb2 = tk.Radiobutton( lf1, text="Two", variable=rbVar, value=2, command=rbCallback) rb2.pack(anchor=tk.W) rb3 = tk.Radiobutton( lf1, text="Three", variable=rbVar, value=3, command=rbCallback) rb3.pack(anchor=tk.W) varLabel = tk.StringVar() tkLabel = tk.Label(lf1, textvariable=varLabel) tkLabel.pack() lf2 = ttk.LabelFrame(tkTop, text="LabelFrame 2") lf2.pack(fill="both", expand="yes") def cb1Callback(): varLabel2.set("Checkbutton 1 clicked: " + str(cb1Var.get())) cb1Var = tk.BooleanVar() cb1 = tk.Checkbutton( lf2, text="Checkbutton 1", width = 50, background='#B0B0B0', anchor=tk.W, variable=cb1Var, command=cb1Callback) cb1.pack() def cb2Callback(): varLabel2.set("Checkbutton 2 clicked: " + cb2Var.get()) cb2Var = tk.StringVar() cb2 = tk.Checkbutton( lf2, text="Checkbutton 2 - ON/OFF", width = 50, background='#C0C0C0', anchor=tk.W, variable=cb2Var, onvalue="ON", offvalue="OFF", command=cb2Callback) cb2.pack() varLabel2 = tk.StringVar() tkLabel2 = tk.Label(lf2, textvariable=varLabel2) tkLabel2.pack() tk.mainloop()
Hello-Python
Friday, December 18, 2015
Python Tkinter: ttk.LabelFrame
Labels:
code example,
tkinter
Python Tkinter: Radiobutton
Example to implement Radiobutton of Python Tkinter.
#for Python 2 #import Tkinter as tk #for Python 3 import tkinter as tk import platform def quit(): global tkTop tkTop.destroy() tkTop = tk.Tk() tkTop.geometry('500x300') tkLabelTop = tk.Label(tkTop, text=" http://hello-python.blogspot.com ") tkLabelTop.pack() strVersion = "running Python version " + platform.python_version() tkLabelVersion = tk.Label(tkTop, text=strVersion) tkLabelVersion.pack() strPlatform = "Platform: " + platform.platform() tkLabelPlatform = tk.Label(tkTop, text=strPlatform) tkLabelPlatform.pack() tkButtonQuit = tk.Button( tkTop, text="Quit", command=quit) tkButtonQuit.pack() def rbCallback(): varLabel.set("Radiobutton clicked: " + str(rbVar.get())) rbVar = tk.IntVar() rb1 = tk.Radiobutton( tkTop, text="One", variable=rbVar, value=1, command=rbCallback) rb1.pack(anchor=tk.W) rb2 = tk.Radiobutton( tkTop, text="Two", variable=rbVar, value=2, command=rbCallback) rb2.pack(anchor=tk.W) rb3 = tk.Radiobutton( tkTop, text="Three", variable=rbVar, value=3, command=rbCallback) rb3.pack(anchor=tk.W) varLabel = tk.StringVar() tkLabel = tk.Label(tkTop, textvariable=varLabel) tkLabel.pack() tk.mainloop()
Labels:
code example,
tkinter
Python Tkinter: Checkbutton
#for Python 2 #import Tkinter as tk #for Python 3 import tkinter as tk import platform def quit(): global tkTop tkTop.destroy() tkTop = tk.Tk() tkTop.geometry('500x300') tkLabelTop = tk.Label(tkTop, text=" http://hello-python.blogspot.com ") tkLabelTop.pack() strVersion = "running Python version " + platform.python_version() tkLabelVersion = tk.Label(tkTop, text=strVersion) tkLabelVersion.pack() strPlatform = "Platform: " + platform.platform() tkLabelPlatform = tk.Label(tkTop, text=strPlatform) tkLabelPlatform.pack() tkButtonQuit = tk.Button( tkTop, text="Quit", command=quit) tkButtonQuit.pack() def cb1Callback(): varLabel.set("Checkbutton 1 clicked: " + str(cb1Var.get())) cb1Var = tk.BooleanVar() cb1 = tk.Checkbutton( tkTop, text="Checkbutton 1", width = 50, background='#B0B0B0', anchor=tk.W, variable=cb1Var, command=cb1Callback) cb1.pack() def cb2Callback(): varLabel.set("Checkbutton 2 clicked: " + cb2Var.get()) cb2Var = tk.StringVar() cb2 = tk.Checkbutton( tkTop, text="Checkbutton 2 - ON/OFF", width = 50, background='#C0C0C0', anchor=tk.W, variable=cb2Var, onvalue="ON", offvalue="OFF", command=cb2Callback) cb2.pack() varLabel = tk.StringVar() tkLabel = tk.Label(tkTop, textvariable=varLabel) tkLabel.pack() tk.mainloop()
Labels:
code example,
tkinter
Thursday, December 17, 2015
Python example: Implement Tab using ttk.Notebook
Python example to implement Tab using ttk.Notebook.
#for Python 2 import Tkinter as tk import ttk #for Python 3 #import tkinter as tk #from tkinter import ttk import platform def quit(): global tkTop tkTop.destroy() tkTop = tk.Tk() tkTop.geometry('500x300') tkLabelTop = tk.Label(tkTop, text=" http://hello-python.blogspot.com ") tkLabelTop.pack() notebook = ttk.Notebook(tkTop) frame1 = ttk.Frame(notebook) frame2 = ttk.Frame(notebook) notebook.add(frame1, text='Frame One') notebook.add(frame2, text='Frame Two') notebook.pack() tkButtonQuit = tk.Button( tkTop, text="Quit", command=quit) tkButtonQuit.pack() tkDummyButton = tk.Button( frame1, text="Dummy Button") tkDummyButton.pack() tkLabel = tk.Label(frame1, text=" Hello Python!") tkLabel.pack() strVersion = "running Python version " + platform.python_version() tkLabelVersion = tk.Label(frame2, text=strVersion) tkLabelVersion.pack() strPlatform = "Platform: " + platform.platform() tkLabelPlatform = tk.Label(frame2, text=strPlatform) tkLabelPlatform.pack() tk.mainloop()
Labels:
code example,
tkinter
Wednesday, December 16, 2015
Python example of using Thread
It's a simple Python example to run code in background thread, to keep GUI responsive.
#tkinter for Python 3.x #Tkinter for Python 2.x import tkinter from threading import Thread import time def quit(): global tkTop tkTop.destroy() def doSomething(): print("Do something") for count in range(5): time.sleep(1) varLabel.set(str(count)) def runThread(): varLabel.set("runThread() called") myThread = Thread(target=doSomething) myThread.start() tkTop = tkinter.Tk() tkTop.geometry('300x200') tkButtonQuit = tkinter.Button( tkTop, text="Quit", command=quit) tkButtonQuit.pack() tkButtonRunThread = tkinter.Button( tkTop, text="Do something in another thread", command=runThread) tkButtonRunThread.pack() tkDummyButton = tkinter.Button( tkTop, text="Dummy Button") tkDummyButton.pack() varLabel = tkinter.StringVar() tkLabel = tkinter.Label(textvariable=varLabel) tkLabel.pack() tkinter.mainloop()
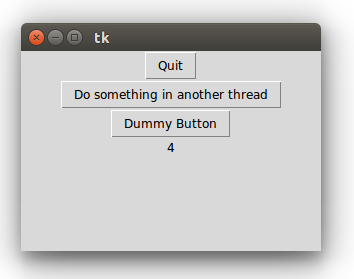
Labels:
code example,
Thread,
tkinter
Friday, March 27, 2015
Python plot sin, cos and tan, using pylab and numpy
import pylab as pl import numpy as np X = np.linspace(0, 2*np.pi, 360, endpoint=True) Y = np.sin(X) Y2 = np.cos(X) pl.plot(X, Y) pl.plot(X, Y2) pl.show()
numpy and matplotlib are needed in this example.
Install for Python 2:
$ sudo apt-get install python-numpy
$ sudo apt-get install python-matplotlib
Install for Python 3:
$ sudo apt-get install python3-numpy
$ sudo apt-get install python3-matplotlib
Labels:
matplotlib,
NumPy
Wednesday, February 11, 2015
Python Tools for Visual Studio
Turn Visual Studio into a powerful Python development environment with Python Tools for Visual Studio.
Python Tools for Visual Studio - free, open-source extension adds all the functionality needed to develop and maintain Python applications in Visual Studio, and deploy to Windows or Linux servers, or to Microsoft Azure.
You can install Python Tools for Visual Studio 2.1 into these versions:
Know more: http://www.visualstudio.com/en-us/explore/python-vs.aspx
This video will help you get up and running with Python in Visual Studio. We will cover installation of PTVS, a Python interpreter, and creating and deploying a project to Azure Websites.
Python Tools for Visual Studio - free, open-source extension adds all the functionality needed to develop and maintain Python applications in Visual Studio, and deploy to Windows or Linux servers, or to Microsoft Azure.
You can install Python Tools for Visual Studio 2.1 into these versions:
- Visual Studio 2013
- Visual Studio Community 2013
- Visual Studio Express 2013 for Web or for Windows Desktop
- Visual Studio 2012 and 2010
Know more: http://www.visualstudio.com/en-us/explore/python-vs.aspx
This video will help you get up and running with Python in Visual Studio. We will cover installation of PTVS, a Python interpreter, and creating and deploying a project to Azure Websites.
Subscribe to:
Posts (Atom)